Python Slots Read Only
You need to allow unlimited read access to a resource when it is not being modified while keeping write access exclusive. Python Slots Read Only, beowulf slot free, t$ pokerstars sell, john poker chips This casino does not have a no deposit bonus at the moment. However, as you have seen in this MegaSlot Casino review, the site has a match bonus which is available to new customers of the casino.
I have been hacking on ways to make lighter-weight Python objects using the__slots__ mechanism that came with Python 2.2 new-style class. Everything

at all!
Here is a simple test case:
import pickle,cPickle
class Test(object):
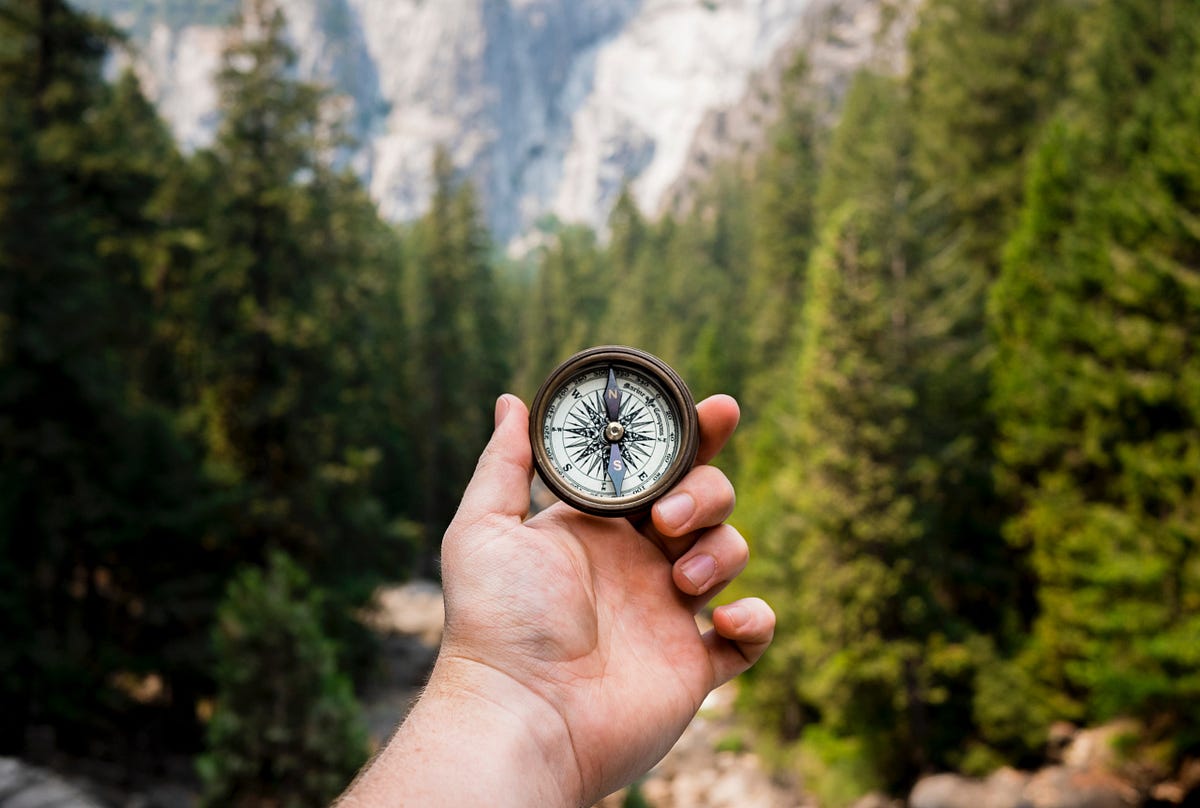
__slots__ = ['x']
def __init__(self):
self.x = 66666
test = Test()
pickle_str = pickle.dumps( test )
cpickle_str = cPickle.dumps( test )
untest = pickle.loads( pickle_str )
untestc = cPickle.loads( cpickle_str )
print untest.x # raises AttributeError
print untextc.x # raises AttributeError
Clearly, this is incorrect behavior. The problem is due a change in object
reflection semantics. Previously (before type-class unification), a
standard Python object instance always contained a __dict__ that listed all
of its attributes. Now, with __slots__, some classes that do store
attributes have no __dict__ or one that only contains what did not fit into
slots.
Unfortunately, there is no trivial way to know what slots a particular class
instance really has. This is because the __slots__ list in classes and
instances can be mutable! Changing these lists _does not_ change the object
layout at all, so I am unsure why they are not stored as tuples and the
'__slots__' attribute is not made read-only. To be pedantic, the C
implementation does have an immutable and canonical list(s) of slots, though

they are well buried within the C extended type implementation.
So, IMHO this bug needs to be fixed in two steps:
First, I propose that class and instance __slots__ read-only and the lists
made immutable. Otherwise, pickle, cPickle, and any others that want to use
reflection will be SOL. There is certainly good precedent in several places
for this change (e.g., __bases__, __mro__, etc.) I can submit a fairly
trivial patch to do so. This change requires Guido's input, since I am
guessing that I am simply not privy to the method, only the madness.
Second, after the first issue is resolved, pickle and cPickle must then be
modified to iterate over an instance's __slots__ (or possibly its class's)
and store any attributes that exist. i.e., some __slots__ can be empty and
thus should not be pickled. I can also whip up patches for this, though I'll
wait to see how the first issue shakes out.
Regards,
-Kevin
PS: There may be another problem when when one class inherits from another
and both have a slot with the same name.
e.g.:
class Test(object):
__slots__ = ['a']
class Test2(Test):
__slots__ = ['a']
test=Test()
test2=Test2()
test2.__class__ = Test
This code results in this error:
Traceback (most recent call last):
File '<stdin>', line 1, in ?
TypeError: __class__ assignment: 'Test' object layout differs from 'Test2'
However, Test2's slot 'a' entirely masks Test's slot 'a'. So, either
there should be some complex attribute access scheme to make both slots
available OR (in my mind, the preferable solution) slots with the same
name can simply be re-used or coalesced. Now that I think about it,
this has implications for pickling objects as well. I'll likely leave
this patch for Guido -- it tickles some fairly hairy bits of typeobject.
Cool stuff, but the rabbit hole just keeps getting deeper and deeper....
--
Kevin Jacobs
The OPAL Group - Enterprise Systems Architect
Voice: (216) 986-0710 x 19 E-mail: jacobs@theopalgroup.com
Fax: (216) 986-0714 WWW: http://www.theopalgroup.com
This tutorial covers the following topic – Python Write File/Read File. It describes the syntax of the writing to a file in Python. Also, it explains how to write to a text file and provides several examples for help.
For writing to a file in Python, you would need a couple of functions such as Open(), Write(), and Read(). All these are built-in Python functions and don’t require a module to import.
There are majorly two types of files you may have to interact with while programming. One is the text file that contains streams of ASCII or UNICODE (UTF-8) characters. Each line ends with a newline (“n”) char, a.k.a. EOL (End of Line).
Another type of file is called binary that contains machine-readable data. It doesn’t have, so-called line as there is no line-ending. Only the application using it would know about its content.
Anyways, this tutorial will strictly tell you to work with the text files only.
Python Write File Explained with Examples
Contents
Let’s begin this tutorial by taking on the first call required to write to a file in Python, i.e., Open().
Open File in Python
You first have to open a file in Python for writing. Python provides the built-in open() function.
The open() function would return a handle to the file if it opened successfully. It takes two arguments, as shown below:
The first argument is the name or path of the file (including file name). For example – sample_log.txt or /Users/john/home/sample_log.txt.
And the second parameter (optional) represents a mode to open the file. The value of the “access_mode” defines the operation you want to perform on it. The default value is the READ only mode.
File Open Modes
It is optional to pass the mode argument. If you don’t set it, then Python uses “r” as the default value for the access mode. It means that Python will open a file for read-only purpose.
However, there are a total of six access modes available in python.
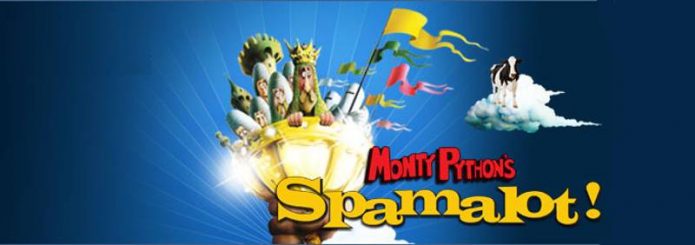
- “r” – It opens a text file for reading. It keeps the offset at the start of the file. If the file is missing, then it raises an I/O error. It is also the default mode.
- “r+” – It opens the file for both READ and WRITE operations. It sets the offset at the start of the file. An I/O error occurs for a non-existent file.
- “w” – It opens a file for writing and overwrites any existing content. The handle remains at the start of the data. If the file doesn’t exist, then it creates one.
- “w+” – It opens the file for both READ and WRITE operations. Rest, it works the same as the “w” mode.
- “a” – It opens a file for writing or creates a new one if the file not found. The handle moves to the end (EOF). It preserves the existing content and inserts data to the end.
- “a+” – It opens the file for both READ and WRITE operations. Rest, it works the same as the “a” mode.
Check out a few examples:
Write File in Python
Python provides two functions to write into a text file: Write() and Writelines().
1. write() – Let’s first use write() for writing to a file in Python. This function puts the given text in a single line.
But, first, open any IDE and create a file named “sample_log.txt” for our test. Don’t make any other changes to it.
Please note – If you try to open a file for reading and it doesn’t exist, then Python will throw the FileNotFoundError exception.
To edit this file from your Python program, we’ve given the following code:
We’ve opened the file in “w” mode, which means to overwrite anything written previously. So, after you open it and see its content, you’ll find the new text in four different lines.
2. writelines() – The writelines() function takes a list of strings as the input and inserts each of them as a separate line in one go. You can check its syntax below:
Append File in Python
You also need to know how to append the new text to an existing file. There are two modes available for this purpose: a and a+.
Whenever you open a file using one of these modes, the file offset is set to the EOF. So, you can write the new content or text next to the existing content.
Let’s understand it with a few lines of code:
We’ll first open a file in “a” mode. If you run this example the first time, then it creates the file.
So far, two lines have been added to the file. The second write operation indicates a successful append.
Now, you’ll see the difference between the “a” and “a+” modes. Let’s try a read operation and see what happens.
The above line of code would fail as the “a” mode doesn’t allow READ. So, close it, open, and then do a read operation.
The output is something like:
Let’s now try appending using the “a+” mode. Check out the below code:
Read File in Python
For reading a text file, Python bundles the following three functions: read(), readline(), and readlines()
1. read() – It reads the given no. of bytes (N) as a string. If no value is given, then it reads the file till the EOF.
2. readline() – It reads the specified no. of bytes (N) as a string from a single line in the file. It restricts to one line per call even if N is more than the bytes available in one line.
3. readlines() – It reads every line presents in the text file and returns them as a list of strings.
It is so easy to use the Python read file functions that you can check yourself. Just type the following code in your IDE or the default Python IDE, i.e., IDLE.
Please note that the Python seek() function is needed to change the position of file offset. It decides the point to read or write in the file. Whenever you do a read/write operation, it moves along.
Close File in Python
File handling in Python starts with opening a file and ends with closing it. It means that you must close a file after you are finished doing the file operations.
Closing a file is a cleanup activity, which means to free up the system resources. It is also essential because you can only open a limited number of file handles.
Also, please note that any attempt to access the file after closing would throw an I/O error. You may have already seen us using it in our previous examples in this post.
With Statement in Python
If you want a cleaner and elegant way to write to a file in Python, then try using the WITH statement. It does the automatic clean up of the system resources like file handles.
Also, it provides out of the box exception handling (Python try-except) while you are working with the files. Check out the following example to see how with statement works.
Python Slots Read Only Online
Working sample code
Below is a full-fledged example that demonstrates the usage of the following functions:
- Python Write file using write() & writelines()
- Python Read file operations using read(), readline(), and readlines()
This Python program generates the following output:
Attempt the Quiz
We’ve now come to the closure of this Read/Write File in Python tutorial. If you have read it from the start to end, then file handling would be on your tips. However, we recommend the following quizzes to attempt.
These are quick questionnaires to test how much knowledge have you retained after reading the above stuff.
Also, you must use these concepts in your projects or can also write some hands-on code to solve real-time problems. It’ll certainly help you grasp faster and remember better.
Python Slots Read Only One
By the way, if you wish to learn Python from scratch to depth, then do read our step by step Python tutorial.
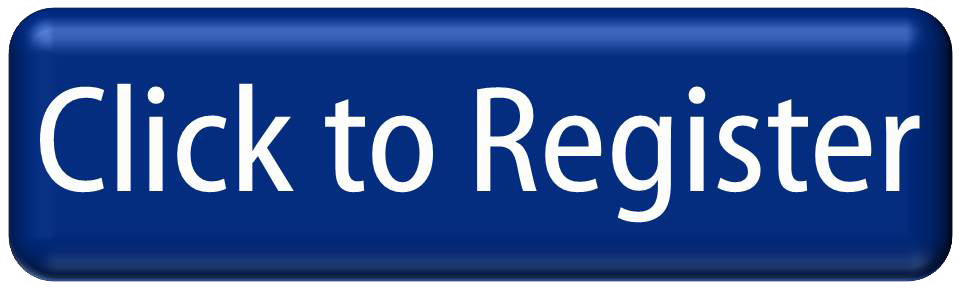